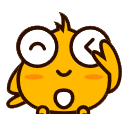
0关注
552
文章
0
收藏
2
次赞
139682
查看
Ta的博客 更多
SpringBoot 五种获取ApplicationContext的方式java中进行日期时间比较的4种方法SpringBoot整合WebSocket实现前后端互推消息CSS怎么画五角星?SpringBoot中RedisUtils工具类配置及直接使用